Populate Selected Item in DropDownList in Edit Mode MVC
Within
last few days I received many questions on this title, so I decided to address
this by a new blog entry. I am going to show you the best possible way to
achieve this using Code First approach. Here is what we are going to achieve.
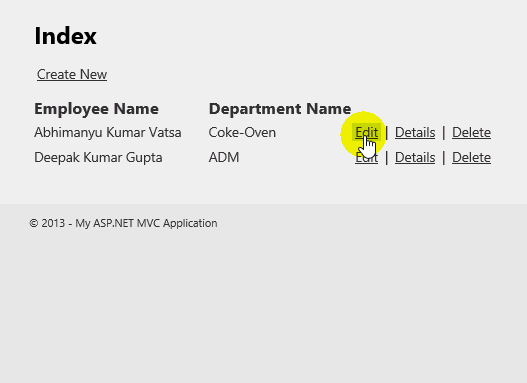
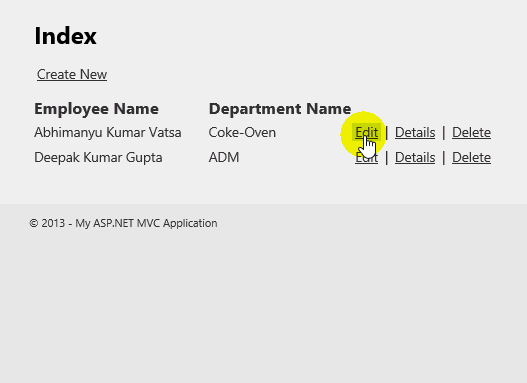
At
very first you should have a better database conceptual model design, if you
fail here will be difficult to achieve this that easily. In the model classes ‘Employee’
and ‘Department’; I’m using minimum properties so that you can understand what
is going on quickly.
Now,
follow the steps from here.
Step 1: Create
conceptual model classes and DbContext
I
am going to create ‘Employee’ and ‘Department’ model classes with the idea in mind,
for each employee in ‘Employee’ table there will be a department in
‘Department’ table.
I
have also decorated both model classes’ properties with appropriate DataAnnotations
& Attributes and also created the possible DbContext for these model
classes.
public class Employee
{
public int EmployeeId { get; set; }
[DisplayName("Employee Name")]
[Required]
public string EmployeeName { get; set; }
[DisplayName("Department Id")]
public int DepartmentId { get; set; }
public virtual Department Department { get; set; }
}
public class Department
{
public int DepartmentId { get; set; }
[DisplayName("Department Name")]
[Required]
public string DepartmentName { get; set; }
public virtual ICollection<Employee> Employees { get; set; }
}
public class CompanyContext : DbContext
{
public DbSet<Employee> Employees { get; set; }
public DbSet<Department> Departments { get; set; }
}
Step 2: Scaffold
Department & Employee Controller
Now,
we have everything need to model our data and save/retrieve it from a database.
And the next step would be creating controller class to enable CRUD.
Right
click on Controller folder to add controller, type ‘DepartmentController’ as
controller name. In scaffolding options area, pick ‘MVC controller with
read/write actions and views, using Entity Framework’ as a Template, pick ‘Department
(MvcApplication1.Models)’ as a Model class and pick ‘CompanyContext
(MvcApplication1.Models)’ as Data context class, now hit Add.
This
will generate all the CRUD views in the application.
Now
repeat this step to generate Employee CRUD views.
Step 3: Enter
Department names and then Employee names
Once
you have CRUD views for both model classes, you can quickly navigate to
department controller and add few records as given below.
Now,
navigate to employee controller and start adding new employee records.
And
now try editing any record. On edit page you will see your previous selection
is still selected.
Step 4: Create
Actions & View
We
have generated our views with the help of scaffolding, so there are few things
generated automatically in controller and view page to support selected item
selected in edit mode.
I
highlighted controller’s actions (GET & POST versions):
public ActionResult Create()
{
ViewBag.DepartmentId = new SelectList(db.Departments, "DepartmentId", "DepartmentName");
return View();
}
[HttpPost]
public ActionResult Create(Employee employee)
{
if (ModelState.IsValid)
{
db.Employees.Add(employee);
db.SaveChanges();
return RedirectToAction("Index");
}
ViewBag.DepartmentId = new SelectList(db.Departments, "DepartmentId", "DepartmentName", employee.DepartmentId);
return View(employee);
}
If
you look in GET version, if you realize SelectList has only three overloads
IEnumerable collection, data value field (what is stored in database) and data
text field (what to display to user on view page).
However,
if you look at POST version, you will find SelectList has four overloads
IEnumerable collection, data value field (what is stored in database), data
text field (what to display to user on view page) and selected values.
Here
is its view page code:
@Html.DropDownList("DepartmentId", "[Select]")
Step 5: Edit
Actions & View
Here,
I also highlighted the controller’s actions (GET & POST versions):
public ActionResult Edit(int id = 0)
{
Employee employee = db.Employees.Find(id);
if (employee == null)
{
return HttpNotFound();
}
ViewBag.DepartmentId = new SelectList(db.Departments, "DepartmentId", "DepartmentName", employee.DepartmentId);
return View(employee);
}
[HttpPost]
public ActionResult Edit(Employee employee)
{
if (ModelState.IsValid)
{
db.Entry(employee).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
ViewBag.DepartmentId = new SelectList(db.Departments, "DepartmentId", "DepartmentName", employee.DepartmentId);
return View(employee);
}
In
both versions, SelectList has four overloads and you can realize now, how it is
working and how simple it is.
Here
is its view page code:
@Html.DropDownList("DepartmentId", "[Select]")
Step 6: Drop Create
Database Always
If
you frequently making changes in your model classes, prefer using following
code to drop and create database for quick demonstrations.
protected void Application_Start()
{
System.Data.Entity.Database.SetInitializer(new DropCreateDatabaseAlways<CompanyContext>());
:::::::::::::
}
Hope
this helps.
Thanks for this useful article. I have managed to obtain the dropdownlist values when I use single parameter table. However, I could not populate multiple ddl from a single Lookup table by using lookup types? Here is what I would like to do. Could you help me please? Thanks in advance...
ReplyDeleteI need to use multiple dropdownlist retrieving data from just ONE LOOKUP table instead of creating another parameter table for each of them. I want to populate multiple dropdownlist on my page retrieving data from a Lookup table in my database and my entity stracture will be simply like that:
Student.cs
...
Student ID
Student Department (ddl 1)
Student Language (ddl 2)
...
Lookup.cs
...
Lookup ID
Lookup Type (i.e. Department, Language)
Lookup Value
...
So, assume that I have Student table and a LOOKUP table containing Department and Language records in it and when populating Department data I will use "Department" and when populating Language data I will use "Language" as Lookup Type. What I would like to do at here to use just a single table for the few parameters instead of using another table for each of them).
Kindest regards.
Any reply???
ReplyDeleteGood job! Help me so much! Thank you!
ReplyDeleteSuperb article, thanks it solved my problem!!!!!!!!!!!
ReplyDeleteGreat Article
ReplyDeleteGreat Article!!!!
ReplyDeleteExcellent Explanation...
ReplyDeleteDynamic DropdownList Step By Step:
http://mamundubd.blogspot.com/2014/03/how-to-create-dynamic-dropdownlist-step.html
You just saved me from over a week long research on this topic.... Nice work... thanks
ReplyDeleteThanks Boss...Your article just works as pain-relief for my long-term pain :D
ReplyDeleteThank You Sir
ReplyDeletegreat article just what i needed i was struggling to save data from a drop-down list to my database.
ReplyDeleteAs you said, in Edit view page code: @Html.DropDownList("DepartmentId", "[Select]").
ReplyDeleteits showing 'select' in dropdown, instead of showing saved gender.
Thank You
ReplyDeleteshowing error System.InvalidOperationException: 'There is no ViewData item of type 'IEnumerable' that has the key 'DDLflatno'.' please help me .
ReplyDelete